Library Fuctions
You can add the library functions by calling the parser with library.js
:
var lang = new octoml('library.js');
Preprocess Tags
Although parsing HTML with RegExps is notoriously difficult, OctoML does have a rudimentary system to recognise HTML tags from the raw text.
Preprocessing tag functions are ran first (hence the name), on the text. Only afterwards is the page parsed as an HTML document. This allows DOM-breaking sections containing CSS, Javascript, Markdown - or anything else.
All library preprocessing tags either remove their content from the page to store elsewhere (such as the <css>
, <less>
or <js>
tags),
or convert the text to parse-able HTML (such as the <markdown>
tag.
Import
You can import other HTML (or OctoML) code from files using the <import>
tag.
Add the filename using the src
attribute.
This is a single tag, so it doesn't need to be closed.
<import src="hello-world.octo">
<div>
<h1>Hello World</h1>
</div>
<h1 bg-danger>Hello World</h1>
Hello World
Templates
Using a template tag, you can import code that wraps around the contents.
The template file is specified with src
The template file can be HTML (or OctoML).
The only addition is the marker %html%
, which will be copied the contents of the template tag.
You can put this marker more than once. To use a different marker, add a marker
<template src="hello-template.octo" color="red">
<p>Template Contents</p>
</template>
<h1 style="color:red">Colored Title</h1>
<p>Template Contents</p>
<hr>
<h1 color="%color%">Colored Title</h1>
%
html
%
<hr>
Colored Title
Template Contents
CSS
The <css>
allows you to place CSS throughout the page (so you can lay out your styles near the elements you're styling).
All CSS is concatenated and appended to the <head>
<css>
.css-example {background:lightgreen;width:100px;height:100px;border-radius:100%;}
</css>
<div class="css-example"></div>
<div class="css-example"></div>
.css-example {background:lightgreen;width:100px;height:100px;border-radius:100%;}
Less
Similarly, you can add Less to your page using the <less>
tag, this will be compiled and added to the CSS.
<less>
@blue: #44F;
.less-example {background: @blue;width:100px;height:100px;}
</less>
<div class="less-example"></div>
<div class="less-example"></div>
.less-example {background:#44F;width:100px;height:100px;}
Javascript
Add javascript or jQuery using the <js>
tags. You can
These will be concatenated, and in the case of jQuery, wrapped in $(function() { ... }
<js require="jquery">
$('#jquery-example').click(function() { alert('clicked!'); });
</js>
<div id="jquery-example">Click Me</div>
<div id="jquery-example">Click Me</div>
$(function() {
$('#jquery-example').click(function() { alert('clicked!'); });
});
Markdown
Using marked.js, OctoML allows you to add markdown to your code by wrapping it in the <markdown>
tag.
<markdown>
# Hello World
[Markdown parsing using marked.js](https://github.com/chjj/marked)
</markdown>
<h1>Hello World</h1><p><a href="https://github.com/chjj/marked">Markdown parsing using marked.js</a></p>
Hello World
Main Tags
After the preprocessing is complete, the page is parsed as an HTML document. This is where the bulk of conversions occur - all the Bootstrap OctoML libraries are Main tags.
There are a handful of libary functions which occur at this point:
Highlight
Automatically highlight code using highlight.js by adding the highlight
attribute to any <code>
tag, or any block element that contains <code>
<pre><code highlight="css">#hello { content: "world"; }</code></pre>
<div highlight="xml">
<pre><code><div id="hello">world</div></code></pre>
</div>
<markdown highlight="javascript">
```
function hello() { console.log('world'); }
```
</markdown>
<pre><code><span class="hljs-id">#hello</span> <span class="hljs-rules">{ <span class="hljs-rule"><span class="hljs-attribute">content</span>:<span class="hljs-value"> <span class="hljs-string">"world"</span></span></span>; }</span></code></pre>
<div highlight="xml">
<pre><code><span class="hljs-tag"><<span class="hljs-title">div</span> <span class="hljs-attribute">id</span>=<span class="hljs-value">"hello"</span>></span>world<span class="hljs-tag"></<span class="hljs-title">div</span>></span></code></pre>
</div>
<pre><code><span class="hljs-function"><span class="hljs-keyword">function</span> <span class="hljs-title">hello</span>(<span class="hljs-params"></span>) </span>{ <span class="hljs-built_in">console</span>.log(<span class="hljs-string">'world'</span>); }
</code></pre>
#hello { content: "world"; }
<div id="hello">world</div>
function hello() { console.log('world'); }
Lorem Ipsum
Generate a random string on Lorem Ipsum text with the single tag <lorem>
.
The default length is 80 words, you can use the short
attribute to get a 10 word snippet.
You can also set any number of words you want using the length
attribute
<lorem>
<br><br>
<lorem length="5">
<p> Ius movet signiferumque ut, ad utamur quaestio vim. Ius id vidit dicant. Mei no melius utroque aliquando. Audiam persequeris at pri. Ne modo omittantur est. Epicurei vituperatoribus cum id, id vim quot aperiri salutandi. Te pro illum utinam, at eos legimus menandri maluisset. Docendi lucilius mel eu, id mea dicta interpretaris. In electram vituperata vis, corpora consulatu vis an, ut sea quot nostrum vulputate. Nam ne iuvaret oportere, dolore oporteat ex vis. Adhuc brute facilis duo ad, eum eu percipitur signiferumque. An mea justo iuvaret, dolore virtute legimus mel ea, eius posse scriptorem nec ea. Ex dicat minim disputando est,.</p>
<br><br>
<p> Vel at everti audiam, ne.</p>
Ius movet signiferumque ut, ad utamur quaestio vim. Ius id vidit dicant. Mei no melius utroque aliquando. Audiam persequeris at pri. Ne modo omittantur est. Epicurei vituperatoribus cum id, id vim quot aperiri salutandi. Te pro illum utinam, at eos legimus menandri maluisset. Docendi lucilius mel eu, id mea dicta interpretaris. In electram vituperata vis, corpora consulatu vis an, ut sea quot nostrum vulputate. Nam ne iuvaret oportere, dolore oporteat ex vis. Adhuc brute facilis duo ad, eum eu percipitur signiferumque. An mea justo iuvaret, dolore virtute legimus mel ea, eius posse scriptorem nec ea. Ex dicat minim disputando est,.
Vel at everti audiam, ne.
You can also add lorem
as an attribute to any tag, and prepend lorem text inside that element.
If you add lorem
to a <tr>
table row, or <ul>
or <ol>
list, you will fill it with <td>
or <li>
s, each with a short Lorem snippet.
Set the number of cells, or list items, with the lorem
attribute.
For a <table>
you must give the size of the table, in the form '3x4' (in this case 3 columns, 4 rows).
The top row will be headers, the remaining rows will be filled with lorem snippets
<p style="color:blue" lorem></p>
<br><br>
<table lorem="3x4"></table>
<br><br>
<ul lorem="5"></ul>
<p style="color:blue"> Mei ei homero iuvaret abhorreant, inermis persecuti at mea. Soluta eripuit eu eam. Eirmod epicuri at his, latine euripidis no ius, cetero nominati conclusionemque sed ea. Ea his vero option persecuti, no nusquam perpetua constituto nec, in aliquip virtute salutandi vis. Ei nusquam laboramus philosophia mel, eam mundi partiendo adversarium at. Te mea docendi volumus neglegentur. Usu scripta iuvaret et, per an iudico feugait. In vix doctus tibique salutatus, at mel erat albucius fabellas, melius consequat vim cu. Vix ex.</p>
<br><br>
<table class="table">
<tbody><tr><th>Heading 1</th><th>Heading 2</th><th>Heading 3</th></tr><tr><td>Eu erant libris noster pro, dictas consetetur mea te.</td><td>Veri illud no mel, illud dolores scriptorem no sit.</td><td>Vel veri soluta quaestio an, no legimus interesset pro.</td></tr>
<tr><td>Id hendrerit mediocritatem ius, nec ad elit vidisse, sed an eleifend deterruisset.</td><td>Ipsum senserit sea id, eam vocent referrentur an, id vivendum delicata ius.</td><td>Qui no oportere definitionem, ex modus quidam adolescens sea.</td></tr>
<tr><td>Ei vis unum altera oblique, ea dolorum adversarium duo.</td><td>Dico alterum referrentur ad nam, duo posse saepe cu.</td><td>Ad dolore delicatissimi mei, solum velit pericula pri ne.</td></tr>
</tbody></table>
<br><br>
<ul><li>No soleat feugiat efficiantur per, his eripuit inermis intellegat ei, sed eu noster labitur aliquando.</li><li>Et mea choro appellantur consequuntur, sit inciderint contentiones ei, cum ne brute feugait consulatu.</li><li>Abhorreant ullamcorper an mei.</li><li>Usu vocent offendit principes eu, posse postulant disputationi ex sit, qui ea mutat brute menandri.</li><li>Quas aeque iudicabit his eu, ex omnis interpretaris vix.</li></ul>
Mei ei homero iuvaret abhorreant, inermis persecuti at mea. Soluta eripuit eu eam. Eirmod epicuri at his, latine euripidis no ius, cetero nominati conclusionemque sed ea. Ea his vero option persecuti, no nusquam perpetua constituto nec, in aliquip virtute salutandi vis. Ei nusquam laboramus philosophia mel, eam mundi partiendo adversarium at. Te mea docendi volumus neglegentur. Usu scripta iuvaret et, per an iudico feugait. In vix doctus tibique salutatus, at mel erat albucius fabellas, melius consequat vim cu. Vix ex.
Heading 1 | Heading 2 | Heading 3 |
---|---|---|
Eu erant libris noster pro, dictas consetetur mea te. | Veri illud no mel, illud dolores scriptorem no sit. | Vel veri soluta quaestio an, no legimus interesset pro. |
Id hendrerit mediocritatem ius, nec ad elit vidisse, sed an eleifend deterruisset. | Ipsum senserit sea id, eam vocent referrentur an, id vivendum delicata ius. | Qui no oportere definitionem, ex modus quidam adolescens sea. |
Ei vis unum altera oblique, ea dolorum adversarium duo. | Dico alterum referrentur ad nam, duo posse saepe cu. | Ad dolore delicatissimi mei, solum velit pericula pri ne. |
- No soleat feugiat efficiantur per, his eripuit inermis intellegat ei, sed eu noster labitur aliquando.
- Et mea choro appellantur consequuntur, sit inciderint contentiones ei, cum ne brute feugait consulatu.
- Abhorreant ullamcorper an mei.
- Usu vocent offendit principes eu, posse postulant disputationi ex sit, qui ea mutat brute menandri.
- Quas aeque iudicabit his eu, ex omnis interpretaris vix.
Clone
Clone another area of of the page using the clone
attribute.
Note this action is done first, before any tags are converted (other than the preprocess tags).
In the example you'll see that the Lorem Ipsum text is different in the clones, since the <lorem>
tag was cloned, then each one was generated separately.
This can be useful for more complex objects (like <carousel>
), which auto-generate their own IDs. By cloning first, each will generate their own unique ID.
Any IDs of elements inside the cloned object will be copied, and cause you trouble.
<div id="clone-example" class="clone-me">
<h3>Hi There</h3>
<lorem length="20">
</div>
<div class="clone-me">Clone multiple objects (added in page order)</div>
<br><hr>#clone-example:<br>
<div clone="#clone-example"></div>
<br><hr>.clone-me<br>
<div clone=".clone-me"></div>
<div id="clone-example" class="clone-me">
<h3>Hi There</h3>
<p> Ex malis nullam pri, eos melius accusam dolores in. Et mea choro appellantur consequuntur, sit inciderint contentiones ei, cum ne.</p>
</div>
<div class="clone-me">Clone multiple objects (added in page order)</div>
<br><hr>#clone-example:<br>
<div>
<h3>Hi There</h3>
<p> Ex malis nullam pri, eos melius accusam dolores in. Et mea choro appellantur consequuntur, sit inciderint contentiones ei, cum ne.</p>
</div>
<br><hr>.clone-me<br>
<div>
<h3>Hi There</h3>
<p> Ex malis nullam pri, eos melius accusam dolores in. Et mea choro appellantur consequuntur, sit inciderint contentiones ei, cum ne.</p>
Clone multiple objects (added in page order)</div>
Hi There
Ex malis nullam pri, eos melius accusam dolores in. Et mea choro appellantur consequuntur, sit inciderint contentiones ei, cum ne.
#clone-example:
Hi There
Ex malis nullam pri, eos melius accusam dolores in. Et mea choro appellantur consequuntur, sit inciderint contentiones ei, cum ne.
.clone-me
Hi There
Ex malis nullam pri, eos melius accusam dolores in. Et mea choro appellantur consequuntur, sit inciderint contentiones ei, cum ne.
Clone multiple objects (added in page order)Populate
Automatically generate links to parts of the page using the populate
attribute.
Provide this with a CSS selector for the items in question, for example, to search for all h2 headings in this article:
<ul populate="article h2"></ul>
<ul><li><a href="#import">Import</a></li><li><a href="#templates">Templates</a></li><li><a href="#css">CSS</a></li><li><a href="#less">Less</a></li><li><a href="#javascript">Javascript</a></li><li><a href="#markdown">Markdown</a></li><li><a href="#highlight">Highlight</a></li><li><a href="#lorem-ipsum">Lorem Ipsum</a></li><li><a href="#clone">Clone</a></li><li><a href="#populate">Populate</a></li><li><a href="#gallery">Gallery</a></li></ul>
Gallery
The <gallery>
tag creates a set of images. You can set with a list of images, or a glob, but appends <img>
s for each entry.
You can supply meaningful alt-text for each image - comma separated - using the alts
attribute, otherwise it will default to a generic 'Image n'.
The gallery tag is a handy shortcut, but also provides context for wrappers like <carousel>
or <thumbnails>
in OctoML/Bootstrap.
<gallery files="img/*.jpg" style="width:150px;height:auto;">
</gallery>
<img src="img/pic1.jpg" alt="Image 1" style="width:150px;height:auto;">
<img src="img/pic10.jpg" alt="Image 2" style="width:150px;height:auto;">
<img src="img/pic2.jpg" alt="Image 3" style="width:150px;height:auto;">
<img src="img/pic3.jpg" alt="Image 4" style="width:150px;height:auto;">
<img src="img/pic4.jpg" alt="Image 5" style="width:150px;height:auto;">
<img src="img/pic5.jpg" alt="Image 6" style="width:150px;height:auto;">
<img src="img/pic6.jpg" alt="Image 7" style="width:150px;height:auto;">
<img src="img/pic7.jpg" alt="Image 8" style="width:150px;height:auto;">
<img src="img/pic8.jpg" alt="Image 9" style="width:150px;height:auto;">
<img src="img/pic9.jpg" alt="Image 10" style="width:150px;height:auto;">
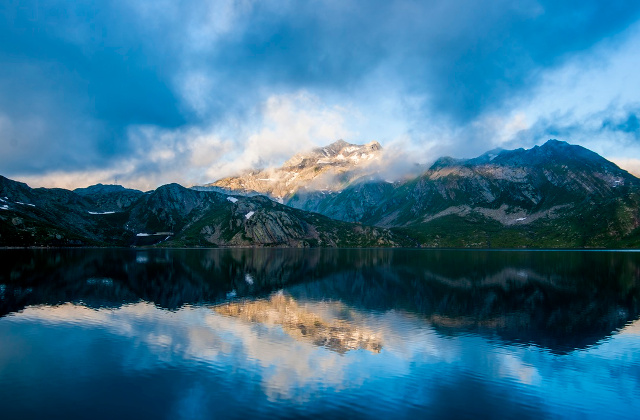
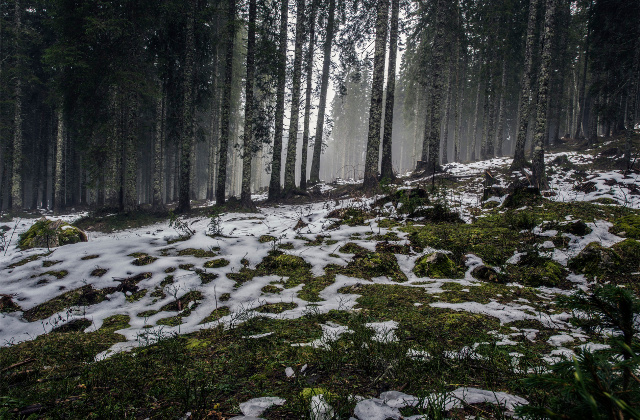
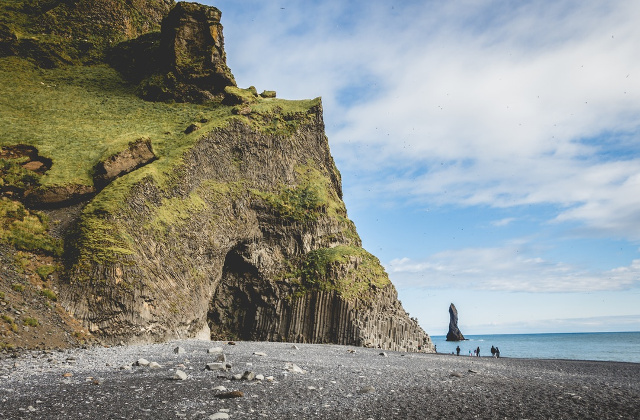
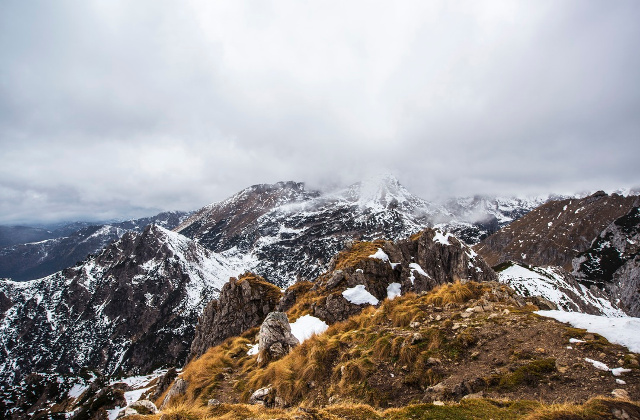
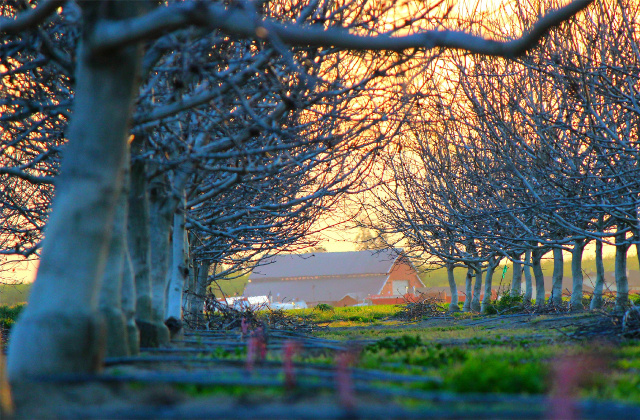
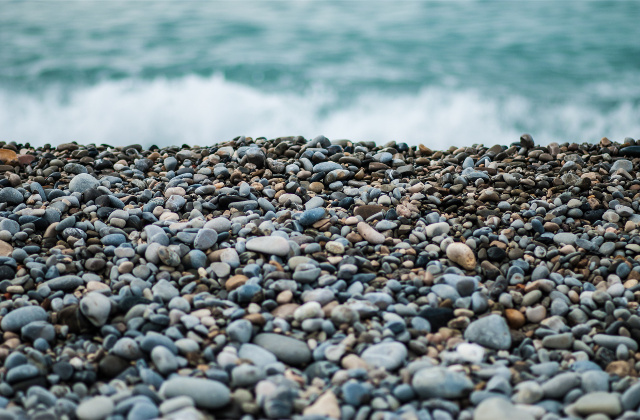
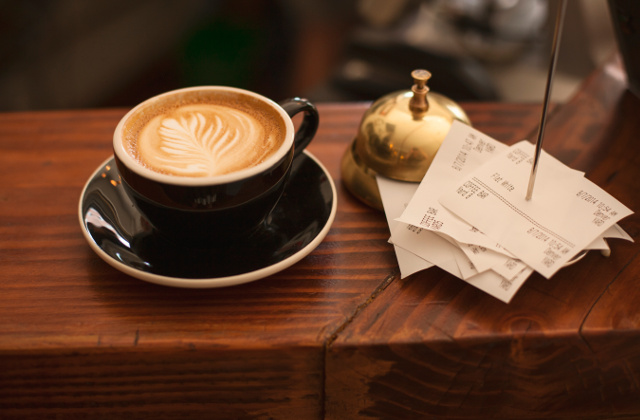
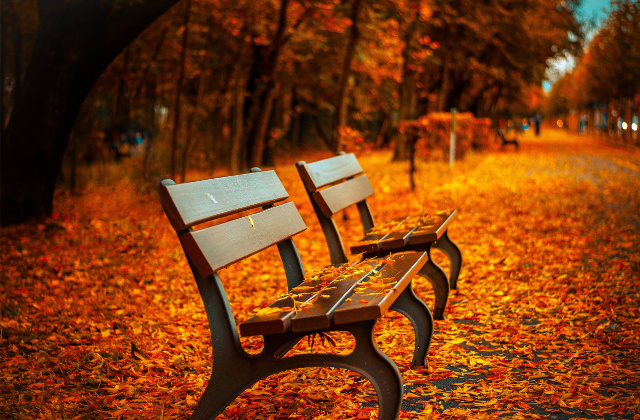
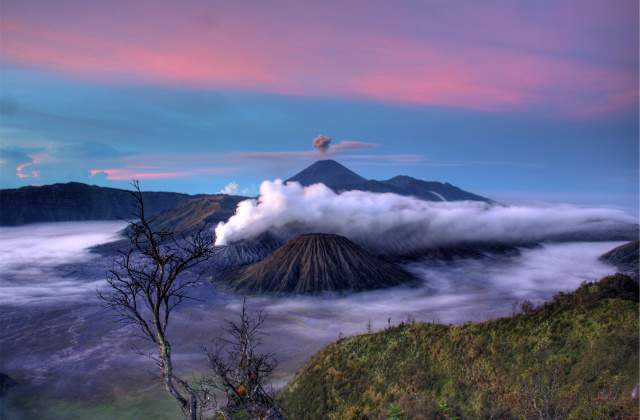
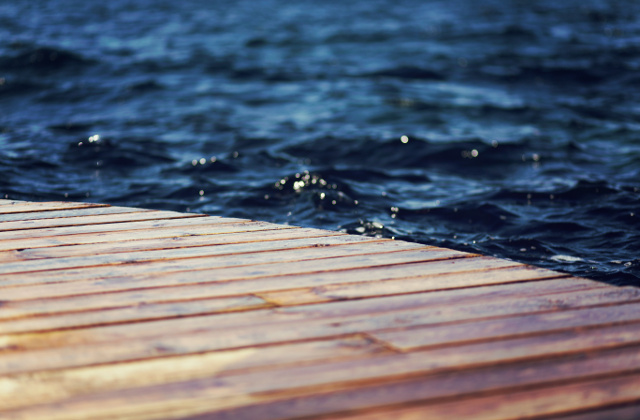
To example above can be achieved:
<gallery pics="pic1-9" extension=".jpg" folder="img"
alts="Image 1,Image 2,Image 3,Image 4,Image 5,Image 6,Image 7,Image 8,Image 9"
style="width:150px;height:auto;">
</gallery>